LitDev Extension API
LDList
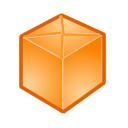
This object provides a way of storing values like an array that reorders itself as items are added or removed.
A list is an efficient array store (much faster than SmallBasic arrays) that can be indexed by integers and perform various other operations.
The indexing is automatically updated (indexed from 1) as the list changes.
Add(listName,value)
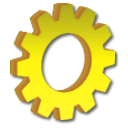
Adds a value to the end of a specified list.
listName The name of the list.
value The value to add.
returns The number of items in the list or -1 on failure.
Append(listName1,listName2)
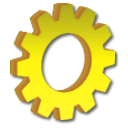
Appends a second list to the end of a first list.
listName1 The name of the first list.
listName2 The name of the second list to append to listName1.
returns The number of items in the list or -1 on failure.
Clear(listName)
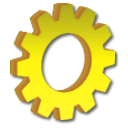
Remove all values from a specified list.
listName The name of the list.
returns The number of items in the list or -1 on failure.
Contains(listName,value)
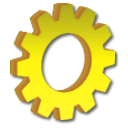
Check if a value is present within the specified list.
listName The name of the list.
value The value to check.
returns "True" or "False".
Copy(listName)
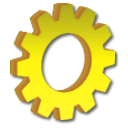
Copy a list.
listName The name of the list.
returns A copy of the list.
Count(listName)
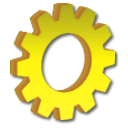
Gets the count of items in the specified list.
listName The name of the list.
returns The number of items in the list or -1 on failure.
CreateFromIndices(sbArray)
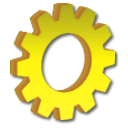
Copy a SmallBasic array indices to a list.
sbArray The SmallBasic array.
returns The created list.
CreateFromValues(sbArray)
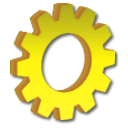
Copy a SmallBasic array to a list.
The array indices are ignored by the list.
sbArray The SmallBasic array.
returns The created list.
Distinct(listName)
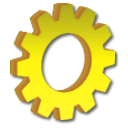
Get a sub list of unique values from the specified list.
The text comparison is case insensitive.
listName The name of the list.
returns The sub list.
Except(listName1,listName2)
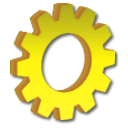
Get a sub list of non-shared values between two specified lists.
The text comparison is case insensitive.
listName1 The name of the first list.
listName2 The name of the second list.
returns The except list.
Find(listName,match,exact)
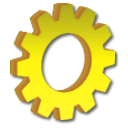
Get a sub list from the specified list where a text match is found.
The text match is case insensitive.
listName The name of the list.
match The match text.
exact An exact match (case insensitive) "True" or the match text is contained in the list "False".
returns The sub list.
FindIndices(listName,match,exact)
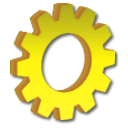
Get a sub list of indices from the specified list where a text match is found.
The text match is case insensitive.
listName The name of the list.
match The match text.
exact An exact match (case insensitive) "True" or the match text is contained in the list "False".
returns The sub list of indices in the list where a match is found.
GetAt(listName,index)
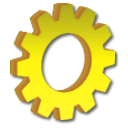
Get a value from a specified list by index (starting from 1).
listName The name of the list.
index The value index to get.
returns The list value.
IndexOf(listName,value)
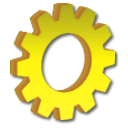
Get the index (starting from 1) of the first occurance of a value from the specified list.
listName The name of the list.
value The value to get index of (0 for not found).
returns The value index or 0.
InsertAt(listName,index,value)
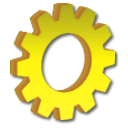
Insert a value in a specified list by index (starting from 1).
listName The name of the list.
index The index to insert at.
value The value to insert.
returns The number of items in the list or -1 on failure.
Intersect(listName1,listName2)
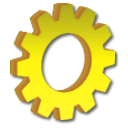
Get a sub list of shared values between two specified lists.
The text comparison is case insensitive.
listName1 The name of the first list.
listName2 The name of the second list.
returns The intersection list.
Print(listName)
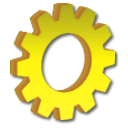
Print a list to the TextWindow.
listName The name of the list.
returns The number of items in the list or -1 on failure.
Read(filePath)
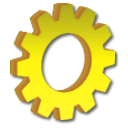
Read a list from a file.
filePath The full path to read the list from.
returns The list if the operation was successful, otherwise "".
Remove(listName,match,exact)
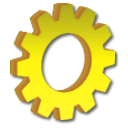
Remove all occurances from the specified list where a text match is found.
The text match is case insensitive.
listName The name of the list.
match The match text.
exact An exact match (case insensitive) "True" or the match text is contained in the list "False".
returns The number of items in the list or -1 on failure.
RemoveAt(listName,index)
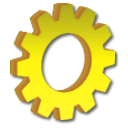
Remove a value from a specified list by index (starting from 1).
listName The name of the list.
index The value index to remove.
returns The number of items in the list or -1 on failure.
Reverse(listName)
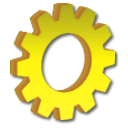
Reverse the order of values in the specified list.
listName The name of the list.
returns The number of items in the list or -1 on failure.
SetAt(listName,index,value)
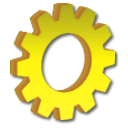
Set (replace) a value in a specified list by index (starting from 1).
listName The name of the list.
index The index to set.
value The value to set.
returns The number of items in the list or -1 on failure.
SortByNumber(listName)
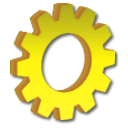
Sort a specified list with values treated as numbers.
All values must be numbers.
listName The name of the list.
returns The number of items in the list or -1 on failure.
SortByText(listName)
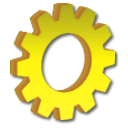
Sort a specified list with values treated as text strings (lexical sort).
The sort is case insensitive.
listName The name of the list.
returns The number of items in the list or -1 on failure.
SubList(listName,start,length)
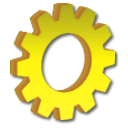
Get a sub list from the specified list.
listName The name of the list.
start The first index of the sub list.
length The length of the sub list.
returns The sub list.
ToArray(listName)
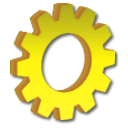
Convert a list to a SmallBasic array.
Not advised for large lists.
listName The name of the list.
returns The Small Basic array.
Union(listName1,listName2)
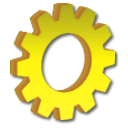
Get a sub list of combined values (duplicates single counted) between two specified lists.
The text comparison is case insensitive.
listName1 The name of the first list.
listName2 The name of the second list.
returns The union list.
Write(listName,filePath,append)
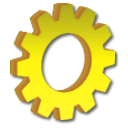
Save a list to file.
One line per list value is used.
listName The name of the list.
filePath The full path to save the list to.
append Append to end of existing file "True" or create a new file "False".
returns The number of items in the list or -1 on failure.