LitDev Extension API
LDArray
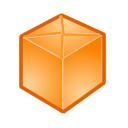
A 1-Dimensional array method that is much faster than the standard SmallBasic arrays.
Useful for arrays with greater than 100 to 1000 elements.
An error will result in a return value "FAILED";
Copy
![]() |
CopyNew
![]() |
CopyToSBArray
![]() |
Count
![]() |
Create
![]() |
CreateFromIndices
![]() |
CreateFromValues
![]() |
Delete
![]() |
GetIndex
![]() |
GetValue
![]() |
Load
![]() |
Save
![]() |
Search
![]() |
SetValue
![]() |
Sort
![]() |
SortIndex
![]() |
Copy(array1,array2)
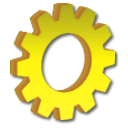
Copy one array to another array.
The dimensions of the 2 arrays must be the same.
array1 The array to copy from.
array2 The array to copy to.
returns "FAILED" or "" for success.
CopyNew(array)
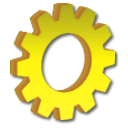
Copy one array to a new array.
array The array to copy.
returns A copy of the array or "FAILED".
CopyToSBArray(array)
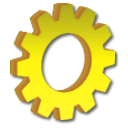
Copy LDArray type to SmallBasic array type.
The reverse operation (SmallBasic to LDArray) isn't possible becuase the SmallBasic indexes are not necessarily contiguous integers.
Note also that a SmallBasic array cannot hold an empty string value, so these will not be copied.
array The array name.
returns The SmallBasic array or "FAILED".
Count(array)
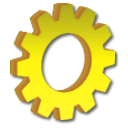
Get the number of non-empty "" elements in the array.
array The array name.
returns The number of non-empty values in the array.
Create(maxSize)
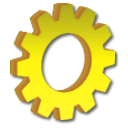
Create a new array (can be used for numbers or character strings).
maxSize The maximum number of elements in the array.
returns The array name or "FAILED".
CreateFromIndices(sbArray)
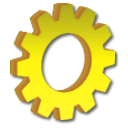
Create a new array from the indices of a Small Basic array.
sbArray The SB array.
returns The new array or "FAILED".
CreateFromValues(sbArray)
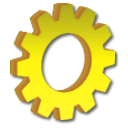
Create a new array from the values of a Small Basic array.
sbArray The SB array.
returns The new array or "FAILED".
Delete(array)
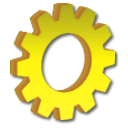
Delete an existing array (not generally required, but can save memory if lots of arrays are created).
array The array name.
returns "FAILED" or "" for success.
GetIndex(sbArray,value)
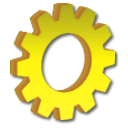
Get the index of the first occurance of a value in an SB array.
sbArray The SB array.
value The value to find.
returns The index of value in the array, "" if not present or "FAILED".
GetValue(array,index)
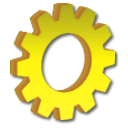
Get value in array.
array The array name.
index The index at which to get the value (indexed starting from 1).
returns The value or "FAILED".
Load(array,fileName)
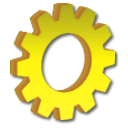
Load an array from a file.
array The array to load the data into.
The array must already exist.
fileName The file path to load the array from.
returns The number of elements loaded.
Save(array,fileName)
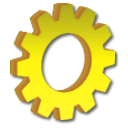
Save an array to a file.
array The array to save.
fileName The file path to save the file to.
returns The number of elements saved.
Search(array,searchstring,match)
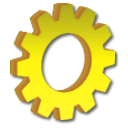
Obtain an array of the indices that have values that contain searchstring.
The search is case in-sensitive.
The input array is unchanged and the match array must be previously created with the same size as the array to check.
array The array to check for matches.
searchstring The string to search for.
match An array to hold the index of matched values.
returns The number of matches found.
SetValue(array,index,value)
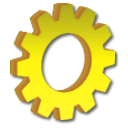
Set value in array.
array The array name.
index The index at which to add the value (indexed starting from 1).
value The value.
returns "FAILED" or "" for success.
Sort(array)
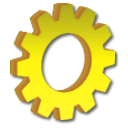
Perform a sort on an LDArray.
By default the sort is by string value, therefore 10 comes before 2.
To sort by number value, all values must be a number (or empty).
Empty values are placed at the end of the sort.
The input array is replaced by the sorted array.
array The array to sort.
returns "FAILED" or "" for success.
SortIndex(array,index)
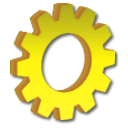
Obtain an array of the indices in sort order of an array.
By default the sort is by string value, therefore 10 comes before 2.
To sort by number value, all values must be a number (or empty).
Empty values are placed at the end of the sort.
The input array is unchanged and the index array must be previously created with the same size as the array to sort.
array The array to obtain an index order sort.
index An array to hold the index order of the sorted array.
returns "FAILED" or "" for success.