LitDev3D Extension API
LD3D |
LD3D
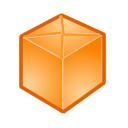
A 3D engine for SmallBasic using the irrlicht engine (http://irrlicht.sourceforge.net/).
The coordinate system is X (left to right) Y (down to up) and Z (front to back).
Move with the arrow keys or WSAD and use Escape to end.
AddAnimatedMesh(mesh,startFrame,endFrame,fps)
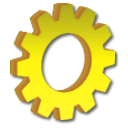
Add a general animated mesh object (*.b3d).
mesh startFrame The starting frame for the animated object.
endFrame The end fame for the animated object.
fps The frames per second for the mesh animation.
returns The added object name.
AddBillBoard(width,height)
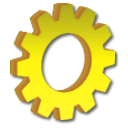
Add a 2D billboard that always faces the camera.
width The width of the billboard.
height The height of the billboard.
returns The added object name.
AddCloudySkydome()
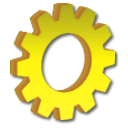
Add a default cloudy skydome.
returns
AddCube()
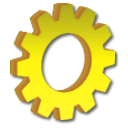
Add a general cube object.
returns The added object name.
AddDungeonMesh(gravity)
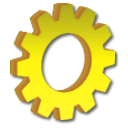
A default Quake mesh.
gravity Gravity of player (0 to fly, 10 for normal gravity).
returns The added object name.
AddFog(colour,density)
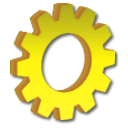
Add a fog effect.
colour The fog colour
density The fog density.
AddLight(X,Y,Z,colour,radius)
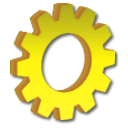
Add a light.
X The light X coordinate.
Y The light Y coordinate.
Z The light Z coordinate.
colour The light colour.
radius The radius of the light.
returns The added object name.
AddMountainSkybox()
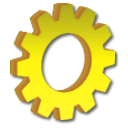
Add a default mountain skybox.
returns
AddMountainTerrain(gravity)
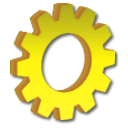
A default terrain.
gravity Gravity of player (0 to fly, 10 for normal gravity).
returns The added object name.
AddNinja(colour)
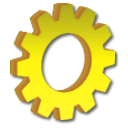
A default nija animated mesh object.
colour "Red" or "Blue"
returns The added object name.
AddQuakeMesh(mesh,gravity)
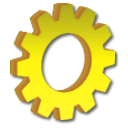
A Quake mesh file within zip mesh file.
mesh The Quake mesh file (*.bsp)
gravity Gravity of player (0 to fly, 10 for normal gravity).
returns The added object name.
AddSkybox(up,down,left,right,front,back)
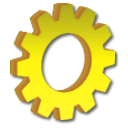
Add a skybox. 6 images are required.
up The up image.
down The down image.
left The left image.
right The right image.
front The front image.
back The back image.
returns
AddSkydome(sky)
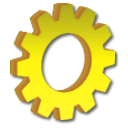
Add a skydome.
sky Image for the skydome (top half of image is above the horizon, the bottom half is below the horizon).
returns
AddSphere()
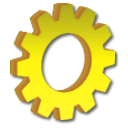
Add a general sphere object.
returns The added object name.
AddTerrain(height,terrain,detail,gravity)
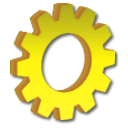
Add a general terrain.
height A heght bitmap (*.bmp)
terrain The main terain image (*.jpg).
detail A detail terrain image (*.jpg).
gravity Gravity of player (0 to fly, 10 for normal gravity).
returns The added object name.
AddZip(name)
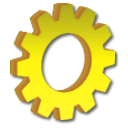
Add a zip for mesh geometries.
name The zip mesh file (*.pk3)
returns
AmbientLight(colour)
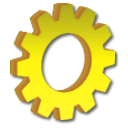
Ambient light level and colour (affects the brightness of objects not directly lit by a light).
colour The ambient colour (default white).
AnimateCircle(name,radius,speed)
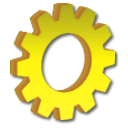
Animate the onject to fly in a horizontal circle about its center with input radius.
name The object name.
radius The radius of the rotation.
speed The speed of the rotation (degrees/second).
AnimateOnTerrainRelative(name,X,Z,time)
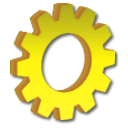
Animate an object relative to its current position while remaining on the ground.
name The object name.
X left right position.
Z near far position.
time the animation time (ms).
returns
AnimateOnTerrainTo(name,X,Z,time)
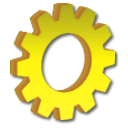
Animate an object to absolute cooordinates while remaining on the ground.
name The object name.
X left right position.
Z near far position.
time the animation time (ms).
returns
AnimateRelative(name,X,Y,Z,time)
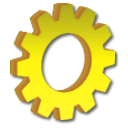
Animate an object relative to its current position.
name The object name.
X left right position.
Y down up position.
Z near far position.
time the animation time (ms).
returns
AnimateRotation(name,X,Y,Z)
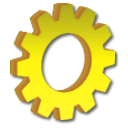
Rotate an object continously.
name The object name.
X Rotation about X axis in degrees per second.
Y Rotation about Y axis in degrees per second.
Z Rotation about Z axis in degrees per second.
returns
AnimateTo(name,X,Y,Z,time)
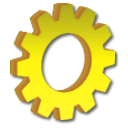
Animate an object to absolute cooordinates.
name The object name.
X left right position.
Y down up position.
Z near far position.
time the animation time (ms).
returns
Click
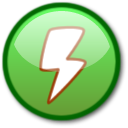
An event that occurs when an object in front of the camera is clicked with the mouse.
The object name is obtained from ClickObject.
ClickObject
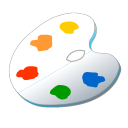
The last object that was clicked.
Collision
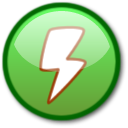
An event that occurs when two objects collide.
To prevent this event from firing multiple times, a short delay (say 100ms) is recommended at the end of this event subroutine.
The object names are obtained from CollisionObject1 and CollisionObject2 in the order they were added.
Only objects added are detected, not for example collision with terrain.
CollisionObject1
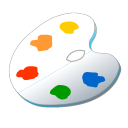
The first object that collided.
CollisionObject2
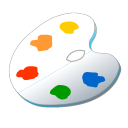
The second object that collided.
ConsoleMessages
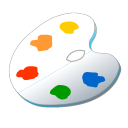
Whether to output errors and warnings to the console "True" or "False".
GetCameraDirection()
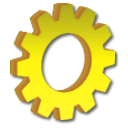
Get the view direction of the camera normalised to unit length.
returns Array of X, Y, Z vector.
GetCameraPosition()
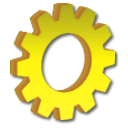
Get the position of the camera.
returns Array of X, Y, Z coordinates.
GetPosition(name)
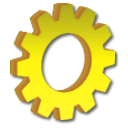
Get the position of an object.
name The object name.
returns Array of X, Y, Z coordinates.
GetTerrainHeight(X,Z)
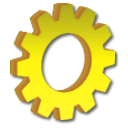
Get the ground level.
X left right position.
Z near far position.
returns The ground level.
GetType(name)
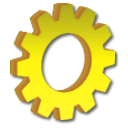
Get a string describing the object type.
name The object name.
returns The object type description.
Remove(name)
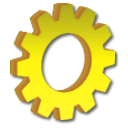
Remove an object.
name The object name.
returns
RemoveAnimators(name)
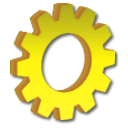
Remove all animations from object.
name The object name.
returns
ResetAllKeyDown()
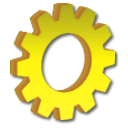
Reset the down state of all keys to "False".
SetCameraProperties(speed,farfield,gravity)
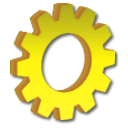
Set some properties of the FPS camera.
speed The speed (default 500).
farfield The farfield view distance (default 10000).
gravity Gravity of player (0 to fly, 10 for normal gravity).
SetGlow(name,colour,radius)
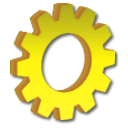
Set an object to glow.
name The object name.
colour The glow colour.
radius The radius of the glow.
returns
SetGravity(name,gravity)
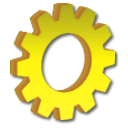
Set gravity for an object
name The object name.
gravity Gravity of object (0 to fly, 10 for normal gravity).
returns
SetPosition(name,X,Y,Z)
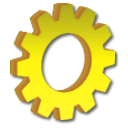
Set the position of an object.
name The object name.
X left right position.
Y down up position.
Z near far position.
returns
SetRotation(name,X,Y,Z)
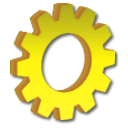
Set the rotation of an object.
name The object name.
X left right rotation (degrees).
Y down up rotation (degrees).
Z near far rotation (degrees).
returns
SetScale(name,X,Y,Z)
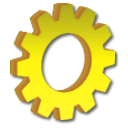
Set the scale of an object.
name The object name.
X left right scale.
Y down up scale.
Z near far scale.
returns
SetShininess(name,shininess)
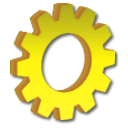
Set size of specular highlights on an object (shininess).
name The object name.
shininess The shininess, 20 is a good value (0.5 to 128).
SetTexture(name,texture)
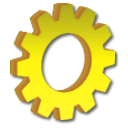
Set the texture on an object.
name The object name.
texture The texture image (*.bmp).
returns
SetType(name,type)
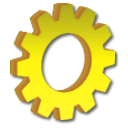
Set a string describing the object type to replace the default.
Useful to detect specific combinations of collisions for example.
name The object name.
type The object type description.
returns
Setup(fullScreen,colour)
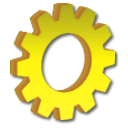
Setup the 3D environment (called before all other LD3D commands).
fullScreen "True" or "False".
colour The background (sky) colour.
returns The added camera object name.
SetVisible(name,visible)
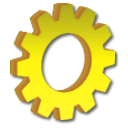
Set the visibility of an object.
name The object name.
visible "True" or "False"
returns
Start()
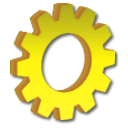
Start LD3D Irrlicht display.
Timer
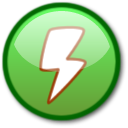
An event that occurs every 100ms to control the game-play.
timerThread()
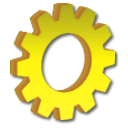
Do the timer callback asynchronously to keep the animation going, and prevent clashing if the callback is not finished before the next call.
WasKeyDown(key)
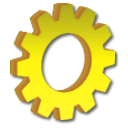
Was the named key down since it was last checked.
A key down state will only be reset to "False" after it is checked explicitly with this command or ResetAllKeyDown() is called.
Only alpha-numeric, function, space, return and shift/control keys supported.
key The key name.
returns "True" or "False"